Unified Modeling Language (UML) is a powerful tool for visualizing, specifying, constructing, and documenting the artifacts of software systems. Among its many features, UML stereotypes play a crucial role in defining the nature and responsibilities of various components within a system. In this article, we will explore UML stereotypes, focusing on three key types: boundary, controller, and entity. We will also discuss their purpose, especially within the context of the Model-View-Controller (MVC) architectural pattern.
What Are UML Stereotypes?
UML stereotypes are a way to extend the vocabulary of UML by adding new semantic meanings to existing elements. They provide a mechanism for categorizing classes and clarifying their roles within a system, allowing developers to communicate design decisions more effectively.
The Three Key Stereotype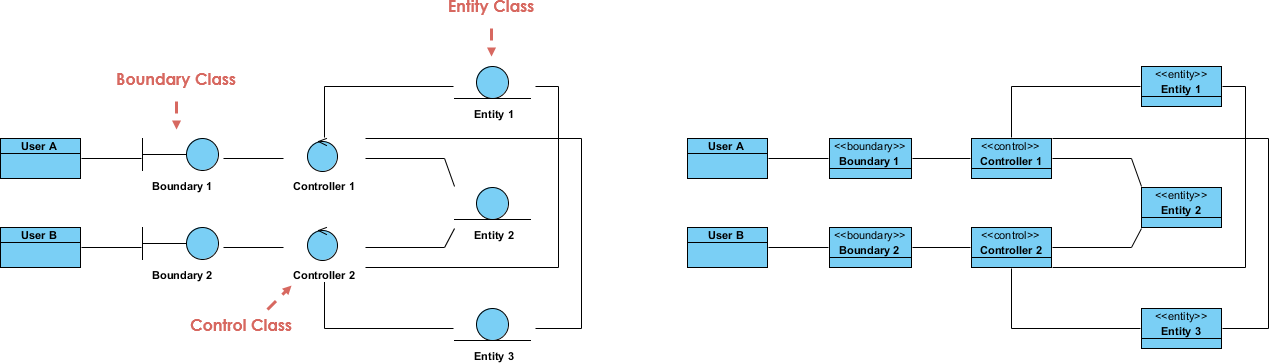
1. Boundary Class
Definition: A boundary class typically represents the user interface or graphical user interface (GUI) components of a system.
Purpose: The boundary class acts as an interface between the users and the system. It receives user inputs and displays outputs, ensuring that the interaction between the system and its users is seamless. By encapsulating the user interface elements, boundary classes help maintain a clear separation between the UI and the underlying business logic.
Example: In the provided class diagram, the boundary classes are illustrated as “Boundary 1” and “Boundary 2,” which interact with users and manage user interactions.
2. Controller Class
Definition: A controller class is responsible for processing user inputs and representing the business logic that connects boundary and entity classes.
Purpose: The controller acts as an intermediary between the user interface and the data objects. It handles the flow of data and commands, ensuring that user requests are processed correctly and that the appropriate responses are generated. By centralizing business logic in controller classes, developers can enhance code maintainability and reusability.
Example: In the diagram, “Controller 1” and “Controller 2” facilitate the interaction between the boundary and entity classes, managing how data is retrieved, manipulated, and stored.
3. Entity Class
Definition: An entity class represents a data object or a persistent data object within the system.
Purpose: Entity classes encapsulate the core data and business rules associated with the system. They are responsible for managing the state and behavior of the data, often corresponding to real-world objects or concepts. By clearly defining entity classes, developers can ensure that the data layer is robust and effectively supports the application’s functionality.
Example: In the class diagram, “Entity 1,” “Entity 2,” and “Entity 3” are depicted as data objects that interact with the boundary and controller classes.
The Role of Stereotypes in MVC Framework
The three stereotypes—boundary, controller, and entity—are essential for implementing the Model-View-Controller (MVC) architectural pattern. This layered approach separates concerns within a software application, leading to improved organization and scalability.
- Model: Represented by entity classes, the model encapsulates the data and business logic.
- View: Represented by boundary classes, the view manages the user interface and displays information to users.
- Controller: The controller orchestrates the communication between the model and the view, processing user inputs and updating the model accordingly.
By utilizing these stereotypes, developers can create a clear structure that enhances understanding and collaboration among team members.
Example of Applying MVC Using UML Stereotypes
In this example, we will illustrate the Model-View-Controller (MVC) architectural pattern using UML stereotypes. We will create a simple application for managing a library system, where users can view books, add new books, and update existing book information. We will represent the components of this MVC architecture using boundary, controller, and entity stereotypes.
Overview of the Application
- Model: Represents the core data structure (books) and business logic.
- View: Represents the user interface components for displaying and interacting with books.
- Controller: Manages user input and updates the model accordingly.
UML Class Diagram
Here’s how the UML class diagram would look:
Description of Each Component
1. Boundary Classes
BookView: This class represents the user interface for displaying the list of books. It includes methods to display books and show messages to the user.
- Methods:
displayBooks()
: Displays the current list of books to the user.showMessage()
: Shows feedback messages based on user actions (e.g., book added successfully).
BookForm: This class represents a form for adding or updating book information.
- Methods:
getBookInput()
: Collects input from the user for book details.showForm()
: Displays the form for user input.
2. Controller Class
BookController: This class handles user interactions and updates the model based on user actions.
- Methods:
addBook()
: Adds a new book to the system by calling methods from theBook
entity.updateBook()
: Updates existing book information.deleteBook()
: Deletes a book from the system.getBooks()
: Retrieves the list of books from the model.
3. Entity Class
Book: This class represents the data structure for a book. It encapsulates the attributes and methods related to book data.
- Attributes:
title
: The title of the book.author
: The author of the book.isbn
: The unique ISBN identifier for the book.
- Methods:
save()
: Saves the book information to the database or data store.update()
: Updates the book information.delete()
: Removes the book from the database.
Modeling a Use Case Scenario in the Library System
To effectively model a use case scenario in the library system, we can leverage the classes defined in our UML design: BookView
, BookForm
, BookController
, and Book
. Below, we will outline how these classes work together in the context of a specific use case: Adding a New Book.
Use Case Scenario: Adding a New Book
Actors Involved
- User: The individual who wants to add a new book to the library.
- System Components:
BookView
: The interface that the user interacts with.BookForm
: The form used to collect book details.BookController
: The component that handles business logic.Book
: The entity representing the book data.
Steps to Model the Use Case
- User Initiates the Process
- The user interacts with the
BookView
to add a new book by clicking a button or link labeled “Add Book.”
- The user interacts with the
- Displaying the Book Form
- The
BookView
invokes theshowForm()
method of theBookForm
class, which presents the user with fields to enter the book’s title, author, and ISBN.
- The
- User Inputs Book Details
- The user fills out the form with the required information and submits it. This action triggers the form to collect the input data.
- Processing the Input
- Upon submission, the
BookForm
calls thegetBookInput()
method to retrieve the entered details (title, author, ISBN). - The
BookForm
then sends this data to theBookController
.
- Upon submission, the
- Adding the Book
- The
BookController
receives the input from theBookForm
and calls thecreateBook()
method on theBook
entity. - Inside the
createBook()
method, a new book object is instantiated with the provided details.
- The
- Persisting the Data
- The
Book
class invokes thesave()
method to store the new book information in the database or data store. - The
Book
class can also handle any necessary validations (e.g., checking for duplicate ISBNs) before saving.
- The
- Confirmation of Success
- Once the book is successfully saved, the
Book
class returns a confirmation to theBookController
. - The
BookController
then calls theshowMessage()
method on theBookView
to inform the user that the book has been added successfully.
- Once the book is successfully saved, the
- User Feedback
- Finally, the
BookView
displays a success message (e.g., “Book added successfully”) to the user, completing the interaction.
- Finally, the
Why Use Case Scenarios Can Be Elaborated by a Sequence Diagram
Use case scenarios provide a high-level overview of how users interact with a system to achieve specific goals. While they effectively outline the functional requirements, sequence diagrams offer a detailed representation of the interactions that occur during these scenarios. Here are several reasons why elaborating use case scenarios with sequence diagrams is beneficial:
1. Visual Representation of Interactions
Sequence diagrams visually depict the flow of messages between various components (actors and system elements) over time. This visual format helps stakeholders quickly grasp how different parts of the system interact during the execution of a use case, making it easier to understand complex processes.
2. Clarification of Roles and Responsibilities
By illustrating the interactions between actors and system components, sequence diagrams clarify the roles and responsibilities of each participant in a use case. This ensures that everyone involved understands who is responsible for what actions, reducing ambiguity and potential misunderstandings.
3. Detailed Workflow Analysis
Sequence diagrams break down the steps involved in a use case scenario, providing a granular view of the workflow. This detailed analysis helps identify potential bottlenecks, redundant processes, or areas for improvement, which can enhance the overall efficiency of the system.
4. Identification of Preconditions and Postconditions
While use case scenarios outline what happens at a high level, sequence diagrams can specify preconditions (what must be true before the scenario begins) and postconditions (the state of the system after the scenario concludes). This information is crucial for understanding the context of the interactions and for ensuring that all necessary conditions are met.
5. Facilitation of System Design and Development
Sequence diagrams serve as a blueprint for developers by providing clear guidance on how various components should interact. This can inform the design of interfaces, methods, and data flows, ensuring that the implementation aligns closely with the intended functionality outlined in the use case scenario.
6. Improved Communication Among Stakeholders
Combining use case scenarios with sequence diagrams enhances communication among stakeholders, including business analysts, developers, and project managers. The visual nature of sequence diagrams makes it easier to convey ideas and gather feedback, fostering collaboration and ensuring that everyone is on the same page.
7. Validation and Verification of Requirements
Sequence diagrams can be used to validate and verify the requirements captured in use case scenarios. By mapping out the interactions, teams can confirm that all necessary functionalities are included and that the system behaves as expected under different conditions. This helps catch potential issues early in the development process.
8. Support for Future Changes and Enhancements
As the system evolves, use case scenarios might need to be updated to reflect new functionalities or changes in requirements. Sequence diagrams provide a detailed view of the current interactions, making it easier to assess the impact of changes and to implement enhancements without disrupting existing functionality.
Class Interactions Summary
- BookView: Manages the user interface and displays messages.
- BookForm: Collects user input and communicates with the controller.
- BookController: Bridges the user interface and data layer, handling business logic.
- Book: Represents the data model, managing the core attributes and persistence logic.
Elaborating use case scenarios with sequence diagrams significantly enhances understanding, clarity, and communication among stakeholders. By providing a detailed, visual representation of interactions, sequence diagrams help ensure that everyone involved has a comprehensive grasp of how the system should behave, facilitating a more efficient and effective development process. This synergy between use case scenarios and sequence diagrams ultimately leads to better-designed systems that meet user needs and expectations.
Conclusion
UML stereotypes serve as a vital tool in enriching the design and documentation of software systems, particularly within the context of the Model-View-Controller (MVC) architectural pattern. By categorizing classes into boundary, controller, and entity stereotypes, developers can clearly communicate the roles and responsibilities of each component, facilitating a better understanding of the system’s structure.
This structured approach to defining boundary, controller, and entity classes allows for effective management of user interactions, business logic, and data representation. The separation of concerns inherent in the MVC framework not only enhances code maintainability but also fosters collaboration among team members. This clarity simplifies the development and extension of the application, ensuring that future enhancements can be integrated smoothly.
In modeling the use case of adding a new book within the library system, we illustrate how each class interacts and contributes to the overall functionality. This delineation of responsibilities promotes organized code architecture, improving scalability and maintainability. By ensuring that each component can evolve independently while remaining part of a cohesive system, the library application is well-positioned for future growth and adaptation. Ultimately, applying these principles leads to more efficient software design and superior project outcomes.